As of Java 8, Java interfaces can include static and default methods. This page describes how to monitor these types of interface methods.
Note that another Java language feature introduced in Java 8, lambda method interfaces, are not supported by the Java Agent.
Interface Static Methods
The following code snippet shows an example static interface method:
package jdk8;
interface InterfaceForStaticMethod
{
static int sum(int acc, int x)
{
return acc + x;
}
}
CODE
The actual bytecode for the method would reside in the compiled class file, so a class match against the interface class in the Splunk AppDynamics configuration would result in the instrumentation of that class for a business transaction.
To instrument such methods, create a POJO business transaction match rule for the interfaceForStaticMethod
, such as:
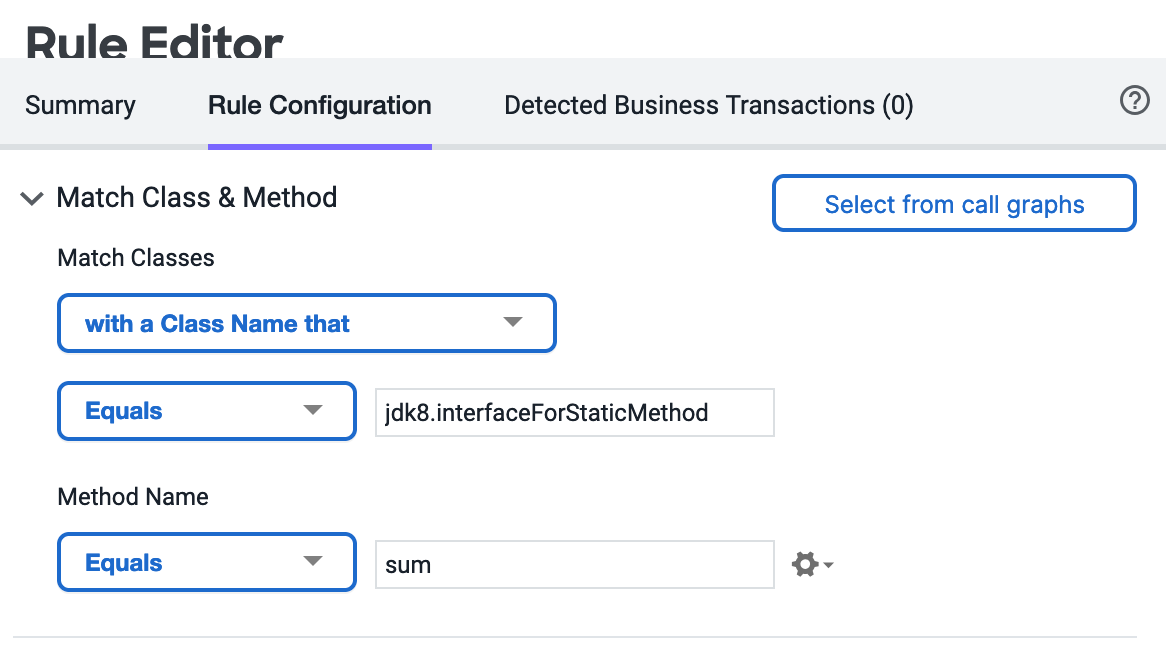
In this case, the rule would match classes with a Class Name of jdk8.InterfaceForStaticMethod
.
Interface Default Methods
Interfaces can also define default methods. As with interface static methods, the bytecode for default methods resides in the compiled class files.
When configuring instrumentation for a default method:
- If the targets are classes that do not override the default method, target the interface via a class match
- If the targets are classes that do override the default method, target the interface via an interface match
Instrument an Interface Default Method Directly
Given the following interface:
package jdk8;
interface InterfaceForConcreteClassDefaultMethod
{
default int sum(int acc, int x)
{
return acc + x;
}
}
CODE
And its implementing class:
package jdk8;
class ClassForDefaultMethod implements InterfaceForConcreteClassDefaultMethod
{
}
CODE
In this example, the ClassForDefaultMethod
does not override the default method. Therefore, you can use a class match against the interface.
Instrument an Overridden Default Method
Given the following interface:
package jdk8;
interface InterfaceForAnonymousClassDefaultMethod
{
default int sum(int acc, int x)
{
return acc + x;
}
}
CODE
And:
InterfaceForAnonymousClassDefaultMethod i = new InterfaceForAnonymousClassDefaultMethod()
{
@Override
public int sum(int acc, int x)
{
return acc + x;
}
};
CODE
Because the method is overridden, the target bytecode
resides in the anonymous class, InterfaceForAnonymousClassDefaultMethod
. So you need to create an interface match POJO business transaction match rule.